I know I'm 7 months late but this is my first code golf answer. I'm looking for some easier coding challenges to take down. I have two answers(one where I tried it without looking at any answers, then one after I looked through some answers.).
First Answer, 62 bytes:
f(N)=\sum_{n=1}^N\left\{\operatorname{mod}(n,10)=2:1,0\right\}
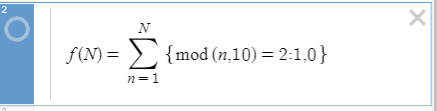
Try it on Desmos!
Explanation:
f(N)= a function taking in an argument of N
\sum_{n=1}^N summation from 1 to N
\left\{ starting piecewise
\operatorname{mod}(n,10)=2: if the remainder of n/10 is 2...
1 sum 1
, otherwise...
0 sum 0
\right\} end piecewise
Not too sure why I can't take out the \left
and \right
for the brackets({
and }
). Theoretically it should work(I've taken out the \left
's and \right
's of all the other "left-right pairs"), but I guess Desmos doesn't allow it.
Second Answer, 20 18 bytes:
Saved two bytes thanks to @golf69
f(N)=floor(.1N+.8)

Try it on Desmos!
Explanation:
My answer is equivalent to f(N)=floor((N+8)/10)
, which is explained in RGS's comment under his answer.