You place a standard die at the origin of a 2D grid that stretches infinitely in every direction. You place the die such that the 1 is facing upwards, the 2 is facing in the negative y direction, and the 3 is facing in the positive x direction, as shown in the figure below:
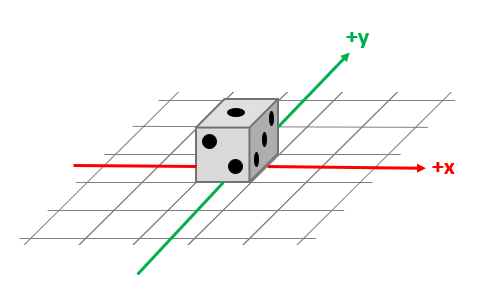
You then proceed to execute a series of moves with the die by rotating it 90 degrees in the direction of motion. For example, if you were to first rotate the die in the negative x direction, a 3 would be upwards, the 2 would be facing in the negative y direction, and a 6 would be facing in the positive x direction.
The series of moves +y, +y, +x, +x, -y
is shown in the figure below, along with the net of the die for clarification (sometimes the net is called a 'right-handed die').
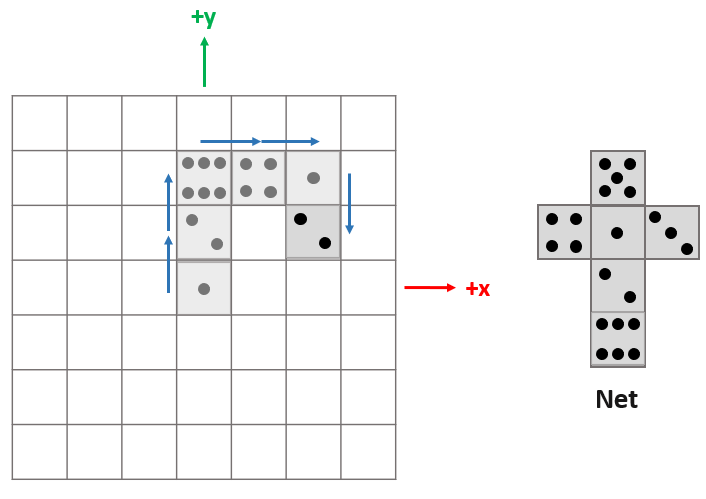
We then proceed to read off the top face of the die after every move. In this case it would read 2, 6, 4, 1, 2
, which we call a dice path. Note we do not include the top face of the die in its initial position, but it is always 1.
If the path of the die is such that it returns to the square it started on at the end of its movement, we call this a dice path that returns to the origin.
Challenge
Given a nonempty dice path as input (in a list or any other reasonable format), print a truthy value if the dice path returns to the origin, and a falsy value otherwise. Note that:
- The truthy values and falsy values you output do not have to be consistent, but you can't swap them (eg. output a falsy value for a path that returns to the origin and a truthy value otherwise)
- The input will be well formed and represent a valid dice path.
- There is no limit to how far the die can stray from the origin.
Test Cases
Path -> Output
2,1 -> true
3,1 -> true
5,4,1,5 -> true
2,4,1,2 -> true
4,2,4,1 -> true
2,4,6,2,4,6,5,4 -> true
2,4,5,1,4,5,3,6,5,1 -> true
5,6,2,3,5,4,6,3,1,5,6,2 -> true
2,4,1,3,5,1,3,5,6,3,5,6,4,5,6,2 -> true
2 -> false
4,5 -> false
5,1,2 -> false
5,6,2,1 -> false
5,4,6,5,4,6 -> false
5,6,4,1,5,4,2,6,5,4 -> false
5,1,2,1,5,6,5,1,2,6,4 -> false
4,6,3,1,5,6,2,1,3,6,4,1 -> false
Scoring
Shortest code in bytes wins.
is_not_prime
. \$\endgroup\$