[Wordle][1] is a [daily online word game][2] that has received [considerable attention recently][3]. The object is to guess a secret word in the fewest attempts. Consider the following instance of the game: 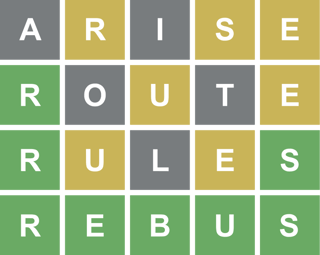 The secret word is `rebus`, and the player's first guess was `arise`. The letters `r`, `s`, and `e` are displayed in yellow to indicate that these letters appear in the secret word, but not at these locations. Meanwhile, the letters in gray do not appear in the secret word at all. The player uses this information to formulate a second guess: `route`. Here, the `r` appears in green, indicating that `r` appears in the secret word at this location. Notice that each guess is an English word. A comment on duplicated letters: If the first guess above were `river`, then the first `r` would appear in green, but the second `r` would appear in gray. In general, the duplicated letters are only highlighted if they are duplicated in the solution, and letters appear in green whenever possible. In this challenge, you will write code that plays Wordle as well as possible. The following link contains a curated list of 2315 common 5-letter words: [words.txt][4] Notably, every Wordle solution to date appears on this list, and I suspect future solutions will, too. Your code will play Wordle by iteratively selecting words from the above `.txt` file and receiving Wordle-style feedback on which letters appear in the secret word. To evaluate your code, play this game 2315 times (each time using a different secret word from the above `.txt` file), and record the number of guesses it took to win. (While the official game terminates after six guesses, in this challenge, you may take arbitrarily many guesses.) In the end, your score will be the histogram of the number of guesses required, arranged in a tuple. For example, `(1,1000,900,414)` is a 4-tuple that indicates that the code solved every word in only 4 guesses, with 414 words requiring all 4 guesses, 900 taking only 3, 1000 taking only 2, and one word being identified after a single guess. Your code must be deterministic, and so the first entry of your score tuple will necessarily be `1`. Scores will be compared in [lexicographic order][5]. That is, shorter tuples are better, and tuples of the same size are first compared in the last entry, then second-to-last entry, etc. For example: `(1,1000,900,414)` < `(1,1000,899,415)` < `(1,999,900,415)` < `(1,998,900,415,1)` Lowest score wins. [1]: https://en.wikipedia.org/wiki/Wordle_(video_game) [2]: https://www.powerlanguage.co.uk/wordle/ [3]: https://www.nytimes.com/2022/01/03/technology/wordle-word-game-creator.html [4]: https://www.dropbox.com/s/p83p1ueoq053xog/words.txt?dl=0 [5]: https://en.wikipedia.org/wiki/Lexicographic_order